
Linked lists are often used to maintain directories of names, or for dynamic memory allocation. Assigns temp node to the current nodes next node current->next. They can be used to implement stacks and queues, as well as other abstract data types. Solution 1: Reverse a linked list using iteration temp current->next. Linked lists are great for quickly adding and deleting nodes. Linked lists may be the second most used data structure, behind arrays. Head pointer: The memory address contained by the head node referencing the first node in the list.
#Reverse a linked list code#
Tail: The last node in a linked list is called the tail. The following chunk of code reverses our linked list starting on root: left root right left.next left.next None while right: temp right.next right.Next: The next node in a sequence, respective to a given list node.
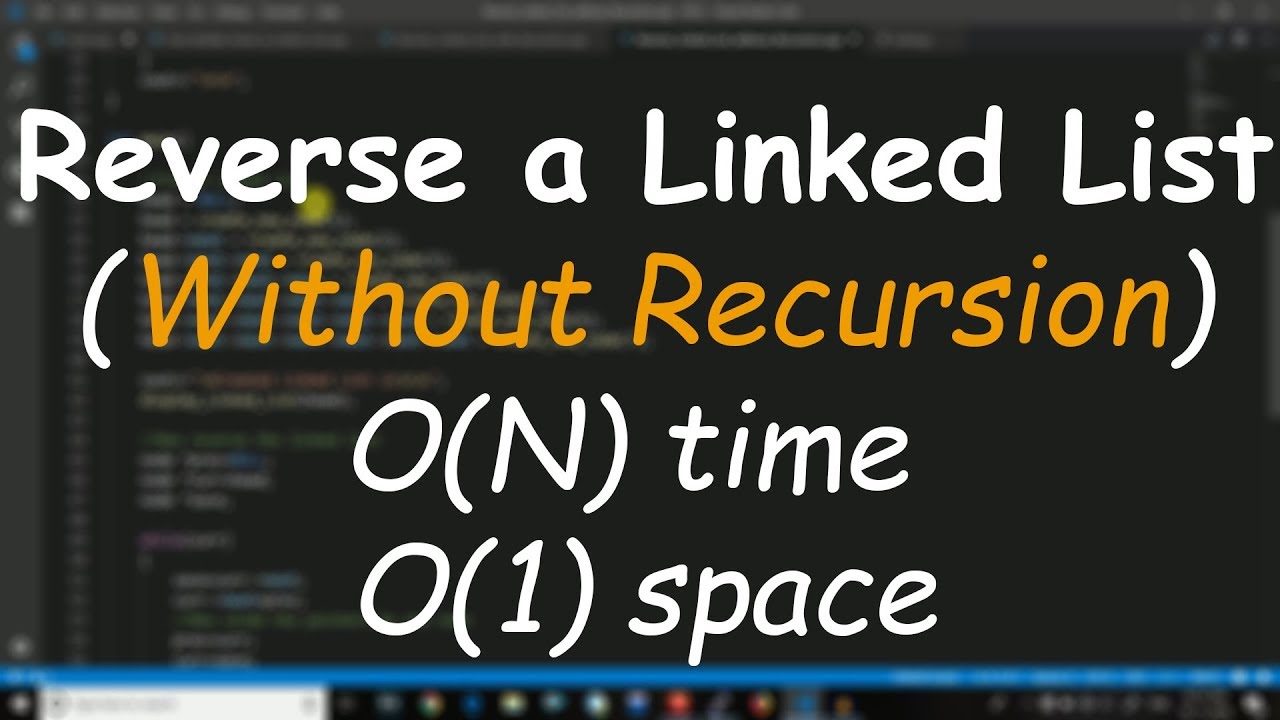

In the case of a singly linked list, the tail node’s pointer is null. A singly linked list is uni-directional while a doubly linked list is bi-directional. A node in a doubly linked list, on the other hand, stores two pointers: one to the next node, and one to the previous node in the sequence. In the case of a singly linked list, nodes only store one pointer to the next node in the sequence. Each node has two components: 1.) A data element and 2.) A pointer containing a memory address to the next node in the list. So, my above refactoring fixed the bug with a bit of serendipity.Linked lists are linear data structures connecting nodes in a sequence. And, I think a for loop can be cleaner: node *Įdit: And, as Vlad mentioned, you don't check for curr being non-null before dereferencing it. Admittedly, with an optimizer, there should be no extra speed penalty.īut, I'd simplify that. Your function is pretty close to optimal.īut, you fetched curr->next twice. To reverse a linked list we will use three pointers: Initialise p to NULL and c equal to head that is it points to the first element. Side note: Curiously, you did an iterative solution for reversal, but did a recursive solutions for create and printList class Node: def init (self, data): self.data data self.next None. First you make a class Node, and initiate a default constructor that will take the value of data in it. Using such a function you could output the list in any stream including a file stream. You can do the following to reverse a singly linked list (I assume your list is singly connected with each other). The steps below describe how an iterative algorithm works: Three-pointers must be initialized, which are called ptrA, ptrB and ptrC. I would declare and define the recursive function the following way FILE * printList( const node *head, FILE *fp )Īnd in main you could write fputc( '\n', printList( head, stdout ) ) A linked list can be reversed in java using two algorithms.

Pay attention to that the parameter of the recursive function printList should be declared with the qualifier const because the list is not changed within the function void printList(cosnt node *head) We need to reverse the list in place by changing the references in a way that the next.
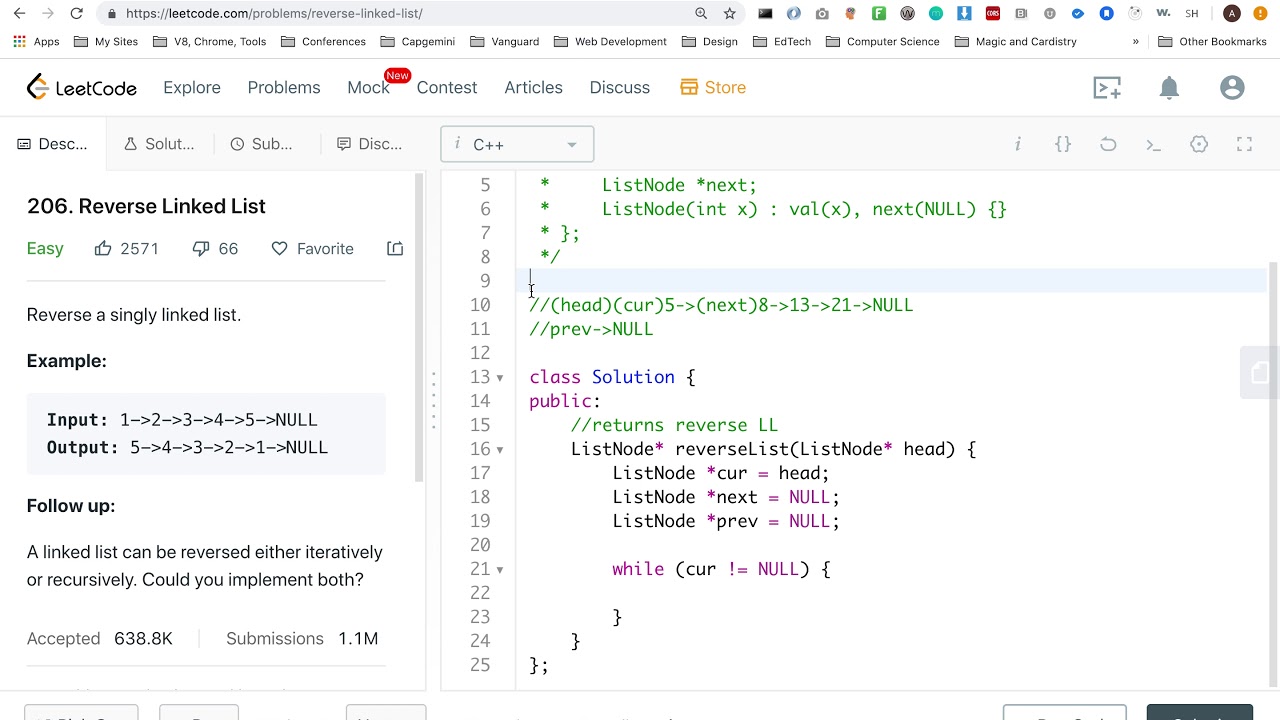
The function can be defined the following way node * reversedList( node *head ) Our goal is to reverse the linked list with a given pointer to the head. So the expression in the while loop while(curr->next != NULL) Reversing a List requires creating three nodes, considering that the list is not empty, which are as follows: tempNode pointing to head, prevNode pointing to. In your function reversedList you do not check whether the passed pointer curr is equal to NULL. The best way to reverse a singly-linked list is to reverse it without invoking undefined behavior Thanks for your suggestions! Have a great day! #include We need to reverse the list by changing the links between nodes. Set the head pointer to the first node only. Link the rest of the list with the first node. Call the recursion for the rest of the linked list to make it in reversed order. I was wondering what the best way to do this would be. Given a pointer to the head node of a linked list, the task is to reverse the linked list. Algorithm: Divide the Linked list into two parts where the first part is the first node and the second part is the remaining list.
#Reverse a linked list how to#
I'm a beginner at programming and I've learnt how to reverse a linked list.īelow is the code I used to make a linked list with random values and reverse it.
